The Ultimate Cheat Sheet On BDD Test Automation with Serenity
This is a true Serenity BDD tutorial. It focuses on open source library Serenity, which helps you to write tests, produce reports and map your automated tests to business requirements.
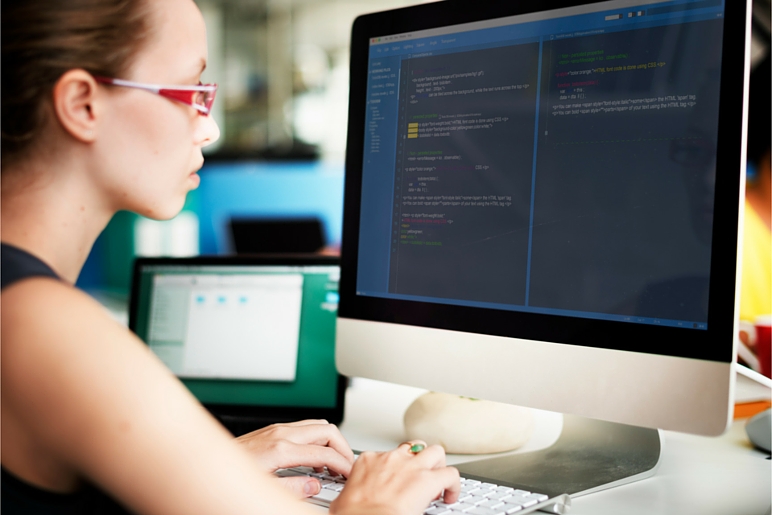
Table of Contents
Let’s run through the basics to clearly understand how it works and how to run and debug your tests.
Table of Contents
What is BDD test?
It’s a behavior-driven development methodology in which an application is specified and designed by describing how it behaves. BDD test offers the ability to enlarge the pool of input and feedback to include business stakeholders and end-users who may not even have software development knowledge. BDD is one of the best options for continuous integration and the Serenity test automation library is the best option for “java-way” implementation.
So, let’s assume that we have a story template:
As a <person (or role)> I want <have some feature> so that <check feature value>
In BDD test, story or feature template looks like scenario:
Given <a scene of the scenario, it is typically something that happened in the past> When <an event, or an action; can be a person interacting with the system, or it can be an event triggered by another system> Then <an expected outcome, or result>
This format is called Gherkin and helps write acceptance criteria in a natural, human-readable form.
BDD test example (feature file):
Feature: Google search In order to find items As a generic user I want to be able to search with Google Scenario: Google search Given I want to search in Google When I search for 'Serenity BDD' Then I should see a link to 'Serenity BDD - Automated Acceptance Testing with Style'
You can use different examples in one scenario by referring to example tables. For instance, the following scenario illustrates how you can verify Google search for different words:
Scenario Outline: Google search multiple Given I want to search in Google When I search for '<search_request>' Then I should see a link to '<search_result>' Examples: | search_request | search_result | | Serenity BDD | Serenity BDD - Automated Acceptance Testing with Style | | Cucumber | Cucumber |
Configuring project
Let’s create a project from scratch by using the Selenium framework as a core of Serenity test automation library.
1) Create project structure (contact us for project source code).
2) Add your feature files:
Create under test/resources feature file:
example.feature
Feature: Google search In order to find items As a generic user I want to be able to search with Google Scenario: Google search Given I want to search in Google When I search for 'Serenity BDD' Then I should see a link to 'Serenity BDD - Automated Acceptance Testing with Style' Scenario Outline: Google search multiple Given I want to search in Google When I search for '<search_request>' Then I should see a link to '<search_result>' Examples: | search_request | search_result | | Serenity BDD | Serenity BDD - Automated Acceptance Testing with Style | | Cucumber | Cucumber |
Organize the feature files in subdirectories according to the requirements. In the following directory structure we have feature definitions for several higher-level features: search and translate
3) Create a BDD testing runner class:
Test runner class runs feature files. If the feature files are not in the same package as the test runner class, you also need to use the @CucumberOptions annotation to provide the root directory where the feature files and step definitions can be found.
Info: File should be in package that we specified in failsafe maven plugin (com.test.serenity.runTests)
GoogleSearchTests.java @RunWith(CucumberWithSerenity.class) @CucumberOptions(features = { "src/test/resources/features" }, glue = { "com.test.serenity.stepDefinitions" }) public class GoogleSearchTests { }
4) Step definitions of our testing tool:
Each line of the Gherkin scenario maps to a method in a Java class, known as a Step Definition. Cucumber annotations like @Given, @When, and @Then match lines in the scenario to Java methods. You should define simple regular expressions to indicate parameters that will be passed into the methods (for example: “I search for ‘something'” will be “I search for ‘(.*)'”):
GoogleSearchStepDefinitions.java public class GoogleSearchStepDefinitions { @Steps GoogleSearchSteps googleSearchSteps; @Given("I want to search in Google") public void iWantToSearchInGoogle() throws Throwable { googleSearchSteps.openGoogleSearchPage(); } @When("I search for '(.*)'") public void iSearchFor(String searchRequest) throws Throwable { googleSearchSteps.searchFor(searchRequest); } @Then("I should see link to '(.*)'") public void iShouldSeeLinkTo(String searchResult) throws Throwable { googleSearchSteps.verifyResult(searchResult); } }
Info: We use @Steps to add a layer of abstraction between the "what" and the "how" of our acceptance tests. Step definitions describe "what" the acceptance test is doing, in clear business domain language. So we say "I search for 'Serenity BDD'", not "user enters 'Serenity BDD' into google search field and clicks on the search button".
File structure for step definitions, steps and running test classes looks like:
5) Steps:
Step Library is a Java class, with methods annotated with the @Step annotation:
GoogleSearchSteps.java public class GoogleSearchSteps { GoogleSearchPage searchPage; GoogleResultsPage resultsPage; @Step public void openGoogleSearchPage() { searchPage.open(); } @Step public void searchFor(String searchRequest) { resultsPage = searchPage.searchFor(searchRequest); } @Step public void verifyResult(String searchResult) { List<String> results = resultsPage.getResultsList(); Assert.assertTrue(results.contains(searchResult)); } }
Step definitions should not be complex, and should focus on working at a single level of abstraction. Step definitions typically operate with web services, databases, or WebDriver page objects. For example, in automated web tests like this one, the step library methods do not call WebDriver directly, but they rather interact with Page Objects.
6) Page Objects:
Page Objects hide the WebDriver implementation details about how elements on a page are accessed and manipulated behind simple methods. Like steps, Page Objects are reusable components that make the tests easier to understand and to maintain.
After extending your page with Page Object, WebDriver instance will be injected into the current page. All you need is to implement WebDriver code that will interact with the page. For example, here is the page object for the Google search page:
@DefaultUrl("https://google.com") public class GoogleSearchPage extends PageObject { @FindBy(id = "lst-ib") private WebElement searchInputField; @WhenPageOpens public void waitUntilGoogleLogoAppears() { $("#hplogo").waitUntilVisible(); } public GoogleResultsPage searchFor(String searchRequest) { element(searchInputField).clear(); element(searchInputField).typeAndEnter(searchRequest); return new GoogleResultsPage(getDriver()); } }
Info: WebDriver method open() opens page according to @DefaultUrl annotation. If @DefaultUrl is missing - WebDriver will open webdriver.base.url from property file.
Info: You can use the $ method to access elements directly using CSS, id or XPath expressions. Or, you may use a member variable annotated with the @FindBy.
Running and debugging
For running your test, use maven command: mvn clean verify
During execution you could monitor logs:
For debugging you should have debug profile in a pom.xml file:
Reports
As for me, reports is the best Serenity feature. You can compile living documentation, combine features and stories in a step-by-step narrative format that includes test data and their execution screenshots.
Main report page shows a wealth of information about tests: count of tests, duration of execution, failed and pending tests, etc.
For example, this page illustrates the test results for our first scenario:
Also, example tables can be a great way to summarize business logic:
And example of report results with errors:
Keep in mind that report will show you what work has been done, and what work is in progress. Any scenario that contains steps without matching step definition methods will appear in the reports as Pending.
Serenity will use feature package structure to group and combine test results for each feature. This way, Serenity can report how well each requirement has been tested, and what requirements have not been tested:
We provide various software testing services including automation testing services to reduce costs, improve security, and boost operational efficiency.